Background and Research
GraphComment is fantastic, but, at least for now, their documentation is pretty scarce and doesn't help much if you're looking to use GraphComment in anything but the most standard scenario. For my part, I wanted to get GraphComment working on the very cool Zvikov theme - but this theme makes use of swiper, a js component that enables you to "swipe" between posts (among other things). This presents two problems:
- As the posts are loaded using js, no js that is included in the post is ever executed
- GraphComment expects there to be a
<div id="graphcomment"></div>
somewhere on the page, but of course if you wish to include more than one commenting areas you will get duplicated ID-s
So after diving into their rudimentary javascript source (thank God it's not minified!) I was able to make some headway.
The self-executing part of their script looks like this:
if (typeof module !== 'undefined') {
module.exports = function(params) {
if (!params.url) throw 'you must provide "url" parameter (full url of your page with GraphComment)';
if (!params.page_title) throw 'you must provide "page_title" parameter (title of your page with GraphComment)';
return graphcomment(params);
};
} else {
if (window.gc_params || window.graphcomment_id) {
var gc_params = window.gc_params || {};
if (window.graphcomment_id) gc_params.graphcomment_id = window.graphcomment_id;
graphcomment(gc_params);
}
}
The key here is that their initialization routine can be called with parameters! Great! Why they don't let their users know in the "documentation" confounds me.
gc_params can contain the following:
var gc_params = {
target: doc.getElementById('graphcomment'), // You can override the default
overlay.z_index
url: '...', // If not provided, this is extracted automatically
page_title: '...', // If not provided, this is extracted automatically
graphcomment_id: '...', // If not provided, this is set to window.graphcomment_id
uid: '...', // Not sure what this is but it is not required
guid: '...', // Not sure what this is but it is not required
identifier: '...', // Not sure what this is but it is extracted from the
// page url if not provided
category: '...', // Not sure what this is but it is not required
readonly: '...', // Not sure what this is but it is not required
inapp: '...', // Not required, seems to be to manually indicate that
// the widget is embedded in a facebook app
publication_date: ..., // Not required
lifetime: ..., // Not required
theme: 'light', // Can be 'light' or 'dark', is determined automatically
// if not set explicitly
sso_public_key: ???, // Not required
sso_data: ???, // Not required
facebook_redirect_after_login: ???, // Not required
twitter_redirect_after_login: ???, // Not required
google_redirect_after_login: ???, // Not required
overlay: false, // Can be set to an object
overlay: {
z_index: 99,
width: 400
},
overlay_direction: 'left', // Not required
overlay_width: 400 // Enter 400 or larger
};
The interesting part here is that you can pass a target as well as the url and page_title manually!
tldr;
Code Injection
Add the following to the "Site Header" box on your code injection page (in your Ghost admin area):
<script type="text/javascript" src="https://graphcomment.com/js/integration.js"></script>
<script type="text/javascript">
(function() {
var postsLoaded = [];
window.postLoaded = function(slug) {
if (!postsLoaded.includes(slug)) {
var target = $('#graphcomment-' + slug);
if (target.length) {
var gc_params = {
graphcomment_id: 'YOUR_GRAPHCOMMENT_ID',
url: target.data("url"),
page_title: target.data("title"),
target: target[0]
};
graphcomment(gc_params);
console.log('GRAPHCOMMENT loaded: #graphcomment-' + slug);
}
else
{
console.log('GRAPHCOMMENT target does not exist: #graphcomment-' + slug);
}
postsLoaded.push(slug);
}
};
})();
</script>
Make sure to replace YOUR_GRAPHCOMMENT_ID
with your actual graphcomment ID. Log into your GraphComments admin panel to get your ID:
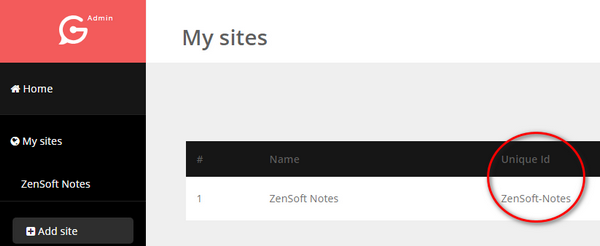
Container DIV
Add a container div somewhere on your page (or .hbs template) as follows:
<div id="graphcomment-{{slug}}" data-title="{{title}}" data-url="{{url absolute="true"}}"></div>
If you use the above, the ID of the div will be set to the slug of your post. You are also setting the page title and url as data-attributes.
Javascript
Now you are able to instantiate as many GraphComment instances on your page as you wish using something like this (paste it in your page directly):
<script type="text/javascript">
(function() {
if (window.postLoaded) {
window.postLoaded('{{slug}}');
}
})();
</script>
Alternatively, call window.postLoaded('slug');
from any script, making sure to pass the appropriate "slug". For example, if your graphcomment div's ID is "graphcomment-polkadots" then you should call window.postLoaded('polkadots');
Best of luck!